using System.Collections.Generic;
namespace MyApplication
{
public static class YieldExample
{
public static IEnumerator<decimal> Go()
{
decimal[] distances = new decimal[] { 67.8M, 0.7M, 6.001M, 63.3M };
foreach (decimal distance in distances)
{
yield return distance;
}
}
}
}
Test like so:
using System.Collections.Generic;
using Microsoft.VisualStudio.TestTools.UnitTesting;
namespace MyApplication.Tests
{
[TestClass]
public class YieldExampleTest
{
[TestMethod]
public void TestMethod()
{
IEnumerator<decimal> decimals = YieldExample.Go();
decimals.MoveNext();
Assert.AreEqual(decimals.Current, 67.8M);
decimals.MoveNext();
Assert.AreEqual(decimals.Current, 0.7M);
decimals.MoveNext();
Assert.AreEqual(decimals.Current, 6.001M);
decimals.MoveNext();
Assert.AreEqual(decimals.Current, 63.3M);
}
}
}
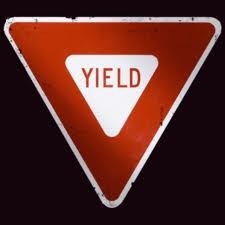
No comments:
Post a Comment