What if you have a working, tested production application that allows you to wring orange juice from an orange like so...
public OrangeJuice MakeOrangeJuice(ref Orange orange)
{
orange.Clean();
OrangeJuice orangeJuice = Wring(orange);
UpdateInventory();
return orangeJuice;
}
...and you want to add new code for squeezing an apple in a similar manner? Maybe apples aren't just like oranges either in terms of workflow. Maybe you should remove the core of an apple before squeezing it to keep the seeds out of the apple juice. (The squeezed apple is gonna break after all. Have you ever seen or used one of those apple core removers?) Would you refactor the existing code like this?
public FruitJuice MakeFruitJuice(bool isToDeseed, ref Fruit fruit)
{
fruit.Clean();
if (isToDeseed)
{
fruit.Deseed();
}
FruitJuice fruitJuice = Wring(fruit);
UpdateInventory();
return fruitJuice;
}
...or, instead of passing in a flag to tweak things in the case of an apple, would you just duplicate the existing logic and then rewrite it for the apple scenario like this?
public OrangeJuice MakeOrangeJuice(ref Orange orange)
{
orange.Clean();
OrangeJuice orangeJuice = Wring(orange);
UpdateInventory();
return orangeJuice;
}
public AppleJuice MakeAppleJuice(ref Apple apple)
{
apple.Clean();
apple.Deseed();
AppleJuice appleJuice = Wring(apple);
UpdateInventory();
return appleJuice;
}
Well, the first approach sure seems better because in the second we repeat ourselves, right?
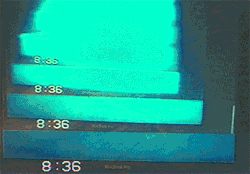
Maybe not. One reason to break the rules is to not break what works. For a team that heavily relieves on unit testing and Selenium tests this wouldn't be a concern, but a traditional testing team would have to test the orange logic that it already blessed as, now, the orange logic has changed. Again, think before you refactor. The testing team can't just test the apple stuff and call it good. Without the Q half of QA you just have "assurance" after all.

No comments:
Post a Comment