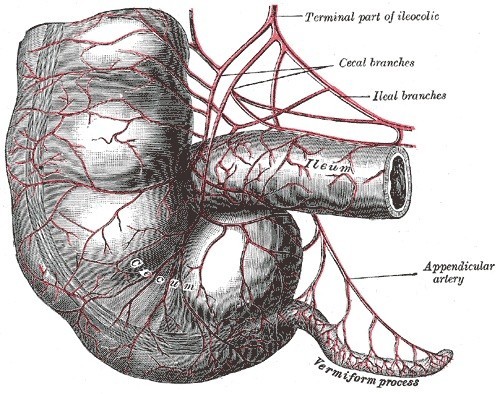
Back for more torture from the book I'm sick of reading, I took it upon myself to read pages 997 to 1004 of Joseph and Ben Albahari's "C# 4.0 in a Nutshell" which is the one and only appendix. The appendix is on C# Keywords and offers a bunch of Webster's Dictionary stuff like this:
- using
- A directive that specifies that types in a particular namespace can be referred to without requiring their fully qualified name types.
- A statement that ensures an object implementing IDisposable is disposed of at the end of the statement's scope.
Uck. If you didn't already know what this means, you'd get nothing from this description. That said, I did take a few things away from this entry. I realized that when I speak of using statements (IDisposable stuff) I am not making an ass of myself as "statements" is the appropriate thing to say. It is using directives in contrast which sort of call out the assemblies which are in scope. All and all, there were four things I learned from the appendix. They are:
- I just mentioned the first thing. Look above.
- The second has to do with signed and unsigned types. As this explains, unsigned numeric types do not have half of their possible values sitting below the zero like a signed counterpart does. int and uint are both 4 bytes in size but the values you may put in an int range from -2,147,483,648 to 2,147,483,647 while the values you may put in a uint are 0 to 4,294,967,295 instead. ulong and ushort are the other two types like uint, and by that I mean they are unsigned. Now that I have read of this in the appendix I vaguely remember reading of it in the meat of the book too.
- Third, I suggest here that one cannot override up to a grandparent. I was wrong! One of the things a sealed class gives you is an assurance that methods decorated with override are not being overridden themselves! You may otherwise override that which is decorated with override as you may override that which is decorated with virtual! Consider these classes:
- namespace Core
{
public class Foo
{
public virtual string DoSomething()
{
return "Foo";
}
}
}
- namespace Core
{
public class Bar : Foo
{
public override string DoSomething()
{
return "Bar";
}
}
}
- namespace Core
{
public class Baz : Bar
{
public override string DoSomething()
{
return "Baz";
}
}
}
using Core;
using Microsoft.VisualStudio.TestTools.UnitTesting;
namespace Tests
{
[TestClass]
public class Inheritance
{
[TestMethod]
public void SimpleInheritance()
{
Bar bar = new Bar();
Foo foo = (Foo)bar;
Assert.AreEqual(foo.DoSomething(), "Bar");
}
[TestMethod]
public void NotSoSimpleInheritance()
{
Baz baz = new Baz();
Foo foo = (Foo)baz;
Assert.AreEqual(foo.DoSomething(), "Baz");
}
[TestMethod]
public void VerboseVersionOfNotSoSimpleInheritance()
{
Baz baz = new Baz();
Bar bar = (Bar)baz;
Foo foo = (Foo)bar;
Assert.AreEqual(foo.DoSomething(), "Baz");
}
}
}
- namespace Core
- Finally, I am also wrong here where I suggest there are no gotos in C#. C# does have a goofy limited goto. It is not what you'd expect from an older language. It is in fact a just way to jump around in a case statement or in other circumstances to do something comparably nasty. Observe:
namespace Core
{
public static class Qux
{
public static int DoSomething(int variable)
{
switch (variable)
{
case(13):
variable = variable + 88;
break;
case(42):
variable = variable + 99;
goto case(13);
break;
default:
break;
}
return variable;
}
}
}
Now behold this test which passes.
using Core;
using Microsoft.VisualStudio.TestTools.UnitTesting;
namespace Tests
{
[TestClass]
public class GoTo
{
[TestMethod]
public void JumpJump()
{
Assert.AreEqual(Qux.DoSomething(7), 7);
Assert.AreEqual(Qux.DoSomething(13), 101);
Assert.AreEqual(Qux.DoSomething(42), 229);
}
}
}
I thought I was done with "C# 4.0 in a Nutshell" last year, but it turns out it still had some things to teach me. This appendix is not just a useless organ where your body stashes the bubblegum you swallow (per urban myth).
No comments:
Post a Comment