I have an idea for a geek movie parody. It will be called "James and the Giant foreach" (instead of "James and the Giant Peach") and it will be about a developer named James who one day, while working, finds a giant foreach loop. Feeling brave, our hero, James, ventures inside and within the foreach he finds... wait of it... a bunch of bugs!
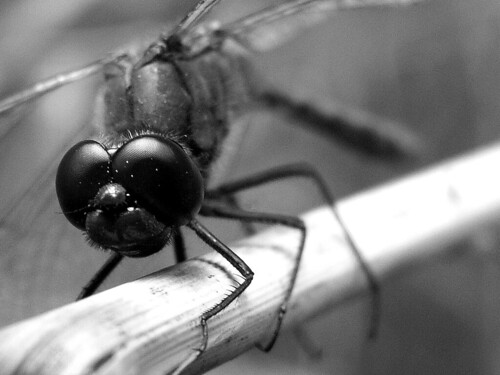
Back to real life: Joel Holder is asserting the foreach is old school and should be stepped away from where possible. An example:
var bars = foos.Where(foo => foo.IsOk).Select(foo => new Bar() {FooName = foo.Name, BarInteger = foo.SecondInteger}).ToList();
The copy above in white may be used to replace the copy below in black if one also uses the System.Linq namespace.
using System.Collections.Generic;
using Microsoft.VisualStudio.TestTools.UnitTesting;
using MyApp.Helpers;
namespace MyApp.Tests
{
[TestClass]
public class Whatever
{
[TestMethod]
public void foreach_versus_select()
{
Foo uno = new Foo
{
Name = "uno",
IsOk = true,
FirstInteger = 88,
SecondInteger = 213
};
Foo dos = new Foo
{
Name = "dos",
IsOk = false,
FirstInteger = 123,
SecondInteger = 87
};
Foo tres = new Foo
{
Name = "tres",
IsOk = true,
FirstInteger = 37,
SecondInteger = 56
};
Foo cuatro = new Foo
{
Name = "cuatro",
IsOk = true,
FirstInteger = 253,
SecondInteger = 568
};
List<Foo> foos = new List<Foo> { uno, dos, tres, cuatro };
List<Bar> bars = new List<Bar>();
foreach (Foo foo in foos)
{
if (foo.IsOk)
{
bars.Add(new Bar
{
FooName = foo.Name,
BarInteger = foo.SecondInteger
});
}
}
Assert.AreEqual(bars.Count, 3);
Assert.AreEqual(bars[1].FooName, "tres");
Assert.AreEqual(bars[2].BarInteger, 568);
}
}
}
No comments:
Post a Comment