Alright, the following is pretty straightforward, right? We are going to see the picture of Robert DeNiro if the bar object hanging off the foo object has a baz property hanging off of it that is truthy.
<div *ngIf="foo.bar.baz">
<img src="DeNiro.jpg" />
</div>
If baz is falsey we will not see the picture and if bar just doesn't have a baz property then we will also not see the picture as baz will read as undefined which is, yes, falsey. But what if foo doesn't even have a bar property? What if bar is undefined? Well then the application is gonna crash, right? It will barf red stuff up to the console in Google Chrome Developer Tools. There is a way to prevent a missing bar object from ruining the show like so:
<div *ngIf="foo.bar?.baz">
<img src="DeNiro.jpg" />
</div>
This is kinda like this stuff from C# 6.0. In the C# scenario I think we are just falling over to null if we cannot read forward (rightward) from the question mark, and that made me initially assume the same of Angular 2, but coworkers pointed out to me that undefined will be used instead of null. If bar was explicitly set to null would it be evaluated as null? I'm not sure. This all came up in conversation yesterday because I saw something like this and initially assumed it would blow up if bar were missing...
<div *ngIf="foo.bar?.baz > 7">
<img src="DeNiro.jpg" />
</div>
It turns out that this is completely legitimate. I think undefined is just used in the comparison and maybe that (er, well, null) would lead to a freak out in C# but this isn't C#. You can compare undefined and null to things in JavaScript with greater than and less than signs. Herein it is important to note the distinction between null and undefined. They are both falsey but can nonetheless compare differently.
|
|
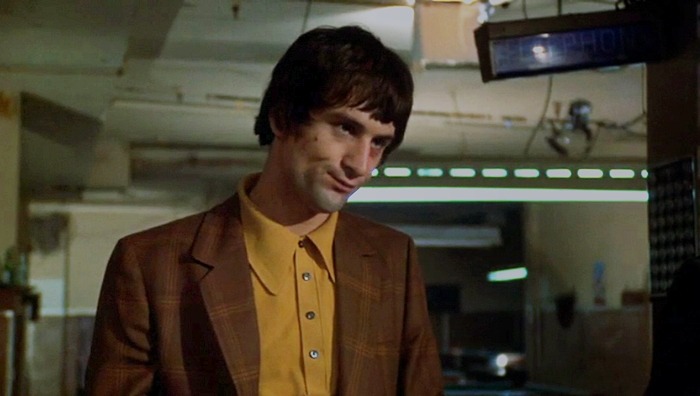
Another place where I've struggled to let go my C# head trash is with private versus public at components. If a field or a method is private in a component it is still accessible from the Angular 2 HTML template. You just can't grab ahold of that thing in the Jasmine/Karma/PhantomJS tests or from other TypeScript objects. It differs of course from web forms code behinds where the counterpart would need public accessibility to get used in the ASP.NET markup.
No comments:
Post a Comment