A web form's code behind:
using System;
namespace WebApplication
{
public partial class _Default : System.Web.UI.Page
{
private Doubler foo;
private Doubler bar;
private Doubler baz;
protected void Page_Load(object sender, EventArgs e)
{
foo = new Doubler(17);
foo.handler += new DoublerEventHandler(OnWhatever);
bar = new Doubler(18);
bar.handler += new DoublerEventHandler(OnWhatever);
baz = new Doubler(19);
baz.handler += new DoublerEventHandler(OnWhatever);
foo.DoubleUp();
bar.DoubleUp();
baz.DoubleUp();
string setBreakPointHere = "whatever";
}
protected static void OnWhatever(Doubler sender, DoublerEventHandlerArgs e)
{
sender.EventValue = e.myNumber;
}
}
}
A delegate:
namespace WebApplication
{
public delegate void DoublerEventHandler(Doubler sender, DoublerEventHandlerArgs
args);
}
A child of EventArgs:
using System;
namespace WebApplication
{
public class DoublerEventHandlerArgs : EventArgs
{
public int myNumber;
}
}
Finally, the Doubler class:
namespace WebApplication
{
public class Doubler
{
public event DoublerEventHandler handler;
public void DoubleUp()
{
DoublerEventHandlerArgs e = new DoublerEventHandlerArgs();
e.myNumber = OriginalValue * 2;
if (handler != null)
{
handler(this, e);
}
}
public Doubler(int originalValue)
{
this.OriginalValue = originalValue;
}
public int OriginalValue;
public int EventValue;
}
}
If I set a breakdown at string setBreakPointHere = "whatever"; and debug, I can tell, by inspecting foo, bar, and baz, that I end up with:
foo | bar | baz | |
OriginalValue | 17 | 18 | 19 |
EventValue | 34 | 36 | 38 |
Note that the logic to do the doubling and the logic to make the assignment to EventValue is split up functionally into two very different places in code. This old school event stuff is more gunk touched on in the delegates chapter of C# 4.0 in a Nutshell. As far as delegate trickery goes, I've seen trickery less clunky. Old school, yo.
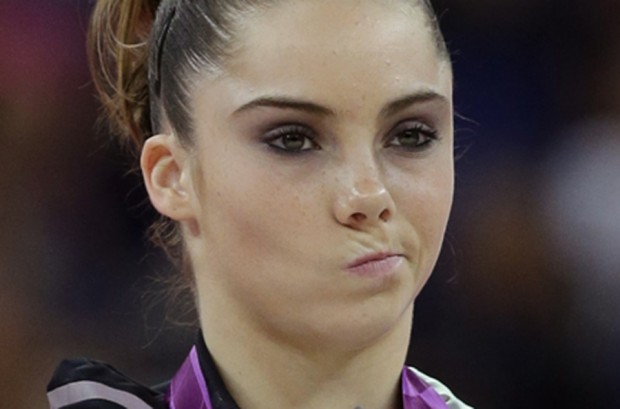
No comments:
Post a Comment