This is a quote by Martin Fowler offered in Jeffrey Palermo's keynote at his MeasureUp conference this year. Depending on your perspective it's either vapid or not vapid, eh? I saw it as vapid first and then I squinted my eyes some. Anyhow, I have five point five pages of hand scribbled notes from a month and a half ago with what I thought the important stuff was. Let me see if I can regurgitate it here. Here's a good start: It was suggested (in the keynote) that your code will be 95% defect free if you undertake and utilize...
![]() |
|
Also in the keynote it was suggested that Scrum works best for something that takes up to one thousand functional points to build and then you're in trouble with it going beyond. At that line-in-the-sand Kanban becomes the better thing and the rollout process for as much should have separate steps for: Requirements discovery, Solution analysis, Key design decisions, Coding, Pull request & review, Integrated developer testing, and Functional testing. Each step needs an inspection process and tests. Every version control repository has one version control counter so it's important to have one application per version control repository. Don't do the old school subversion thing in which you just use a repository like a file share. Bad. The Addison-Wesley book "Continuous Delivery" was recommended. James Chambers talk was a .NET Core "all-you-can-eat buffet" and .NET Core is now open source and available on GitHub and treated as a first class citizen. He went through setting up a new MVC app and slurping down NuGet dependencies. WebBundler will be used instead of Gulp for bundling and minification. In the new paradigm there will be a wwwroot folder and .css and .dll files will get built here. When the web server spins up it will look here first and Startup.cs and Program.cs are the first two files it will find to orient itself. You will now just inherit from "Controller" to make either an MVC Controller or a Web API Controller and if you just use the WhateverController naming convention you don't even have to inherit from Controller. There is a Web.config but project.json is really the new Web.config. Kestrel is the new web server that's not IIS and not tied to Windows. You can stand up the Nginx web server in front of Kestrel in Linuxland and Kestrel is eight to ten times faster than Node.js. Throw in a Docker container to get this working at Amazon. The Program.cs entry point for the application exposes something that CAN be used for something other than the MVC framework. For example:
public static void Main(string[] args)
{
var host = new WebHostBuilder()
.UseKestrel()
.UseContentRoot(Directory.GetCurrentDirectory())
.UseIISIntegration()
.UseStartup<Startup>()
.Build();
host.Run();
}
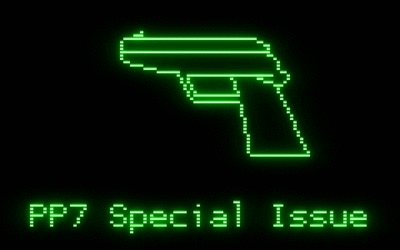
public IConfiguration Configuration {get;} in Startup.cs similarly allows for bringing in the configuration "options" pattern, opening the door to reading a bit of a configuration from AppSettings.json in the name of hydrating static unchanging properties. You're going to have a secrets file that does not get checked into source control. Some other wire-ups are:
builder.AddUserSecrets();
service.AddMvc();
app.UseDeveloperExceptionPage();
So the first of these loops in the secrets file, the second has to do with controller resolvers and other dependency injection stuff for MVC, and the last thing empowers the yellow and red screen of death, but conditionally so for dev and not production. Hmmm... I'm a little over halfway through my notes now. I'm going to take a break from typing and get something to eat. More later.
Addendum 9/9/2016: "Middleware is like Russian Dolls where you open it up, you open it up, you open it up..." was a quote from Simon Timms in his talk about "A sink full of C#" wherein he suggested that semaphores and mutexes require critical thinking. (Wikipedia says "a semaphore is a variable or abstract data type that is used for controlling access, by multiple processes, to a common resource in a concurrent system" and also "software that acts as a bridge between an operating system or database and applications, especially on a network" is the definition I see when I Google middleware.) Things have changed in our space. In "the old days" we had:
public static void Main(string[] args)
{
var thread = new Thread(Whatever);
thread.Start();
Console.ReadLine();
}
result.AsyncWaitHandle is coming back to .NET but the task parallel library is the new way to go. It uses "tasks" which are basically just boxes holding results. The I/O completion port signals back into the framework when a task is complete in this model. There is a distinction between these two...
- public async Task Whatever() {
- public async void Whatever() {
...in that you can wrap them both in a try/catch but the exception will get swallowed in the case of the void. Async doesn't always run on a new thread. It is about using the client efficiently. During the lunch break at MeasureUp a strange video was played to make us glad we work with C# instead of JavaScript which showed off a lot of the wacky idiosyncrasies in JavaScript that makes me put up walls and close my mind rather than embrace the language. Number(true) will give you 1 for example, and stuff like that. Yucky. "foo" + + "bar" gives us fooNaN and 0.1 + 0.2 gives us 0.30000000000000004 and so on. ~~4.2 is the same as Math.round(4.2) in that there is an operator for rounding and you rock the double tilde to go there. Brian named dropped the Fetch Standard of which this suggests: "fetch() allows you to make network requests similar to XMLHttpRequest (XHR). The main difference is that the Fetch API uses Promises, which enables a simpler and cleaner API, avoiding callback hell and having to remember the complex API of XMLHttpRequest." You need to install both node and jspm in Brian's model and some command line commands at npm were:
- jspm init
- jspm install aurelia-framework
Let's see. Some of the nonsense I scribbled down includes: Views in Aurelia are at the root and a template tag wraps everything else. Whatever that means. When you slurp in POCOs from a Web API endpoint at JSON objects, by default the names will swap from PascalCase to camelCase in abandoning the C# naming convention for the JavaScript convention. aurelia.io is the Aurelia homepage. Kyle Nunnery spoke last on making beautiful web APIs using ASP.NET Core. He suggested a good approach is an API first approach. His approach is:
- I'm going to build an API.
- I'm going to build my tooling on top of that.
- I'm going to build other tooling on top of that.
Kyle recommends url-based versioning like so: https://api.yourwebsite.com/v1/llamas ...and initially keeping messaging down to four simple status codes:
200 | OK |
400 | Bad Request |
401 | Unauthorized |
500 | Internal Server Error |
When you use Kestrel you can define options inside the parenthesis at .UseKestrel() as suggested above. Swagger was recommended for documentation.
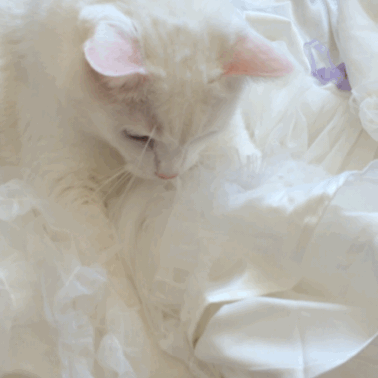
No comments:
Post a Comment